22. 12. 2023
ALERT: This article might be outdated and not fit for your needs. In order to enjoy its updated version, visit Android Guide To: Drawing Text Over Bitmap
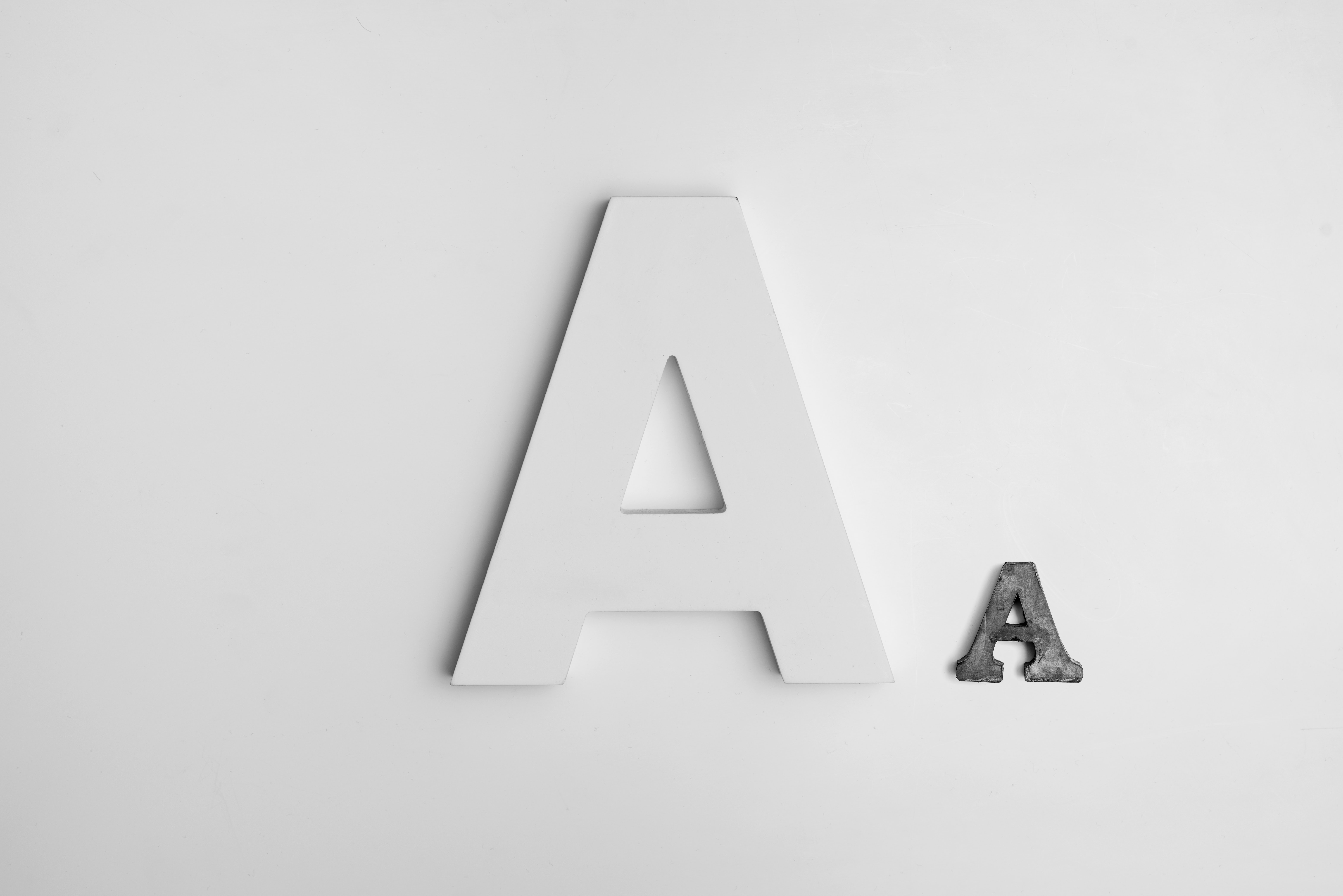
This short article shows you how to draw text on bitmap loaded from resources. You can see also multiline version.
First we read bitmap from resources. This bitmap is immutable, so we create mutable copy. Then we create Paint object and set them text size, color, shadow, etc.
Now we are ready to draw! But where? We need to calculate position of the bottom left corner of drawn text. That’s all. Enjoy!
public Bitmap drawTextToBitmap(Context gContext, int gResId, String gText) { Resources resources = gContext.getResources(); float scale = resources.getDisplayMetrics().density; Bitmap bitmap = BitmapFactory.decodeResource(resources, gResId); android.graphics.Bitmap.Config bitmapConfig = bitmap.getConfig(); // set default bitmap config if none if(bitmapConfig == null) { bitmapConfig = android.graphics.Bitmap.Config.ARGB_8888; } // resource bitmaps are imutable, // so we need to convert it to mutable one bitmap = bitmap.copy(bitmapConfig, true); Canvas canvas = new Canvas(bitmap); // new antialised Paint Paint paint = new Paint(Paint.ANTI_ALIAS_FLAG); // text color - #3D3D3D paint.setColor(Color.rgb(61, 61, 61)); // text size in pixels paint.setTextSize((int) (14 * scale)); // text shadow paint.setShadowLayer(1f, 0f, 1f, Color.WHITE); // draw text to the Canvas center Rect bounds = new Rect(); paint.getTextBounds(gText, 0, gText.length(), bounds); int x = (bitmap.getWidth() - bounds.width())/2; int y = (bitmap.getHeight() + bounds.height())/2; canvas.drawText(gText, x, y, paint); return bitmap; }
EDIT 2013-11-16: Fixed bug in canvas.drawText() call. Variables x and y are no longer multiplied by scale.
EDIT 2015-11-14: Added link to multiline version.
Do you need more detailed help? Book a consultation:
Let’s chat about it
Do you want more?
We share the world of software development on our Instagram. Join us 🙂
We also tend to look for Android dev (iOS as well), but there are also chances for a project manager or a tester. Send us an e-mail: [email protected].